back to indexIntro to APIs in Python - API Series #1
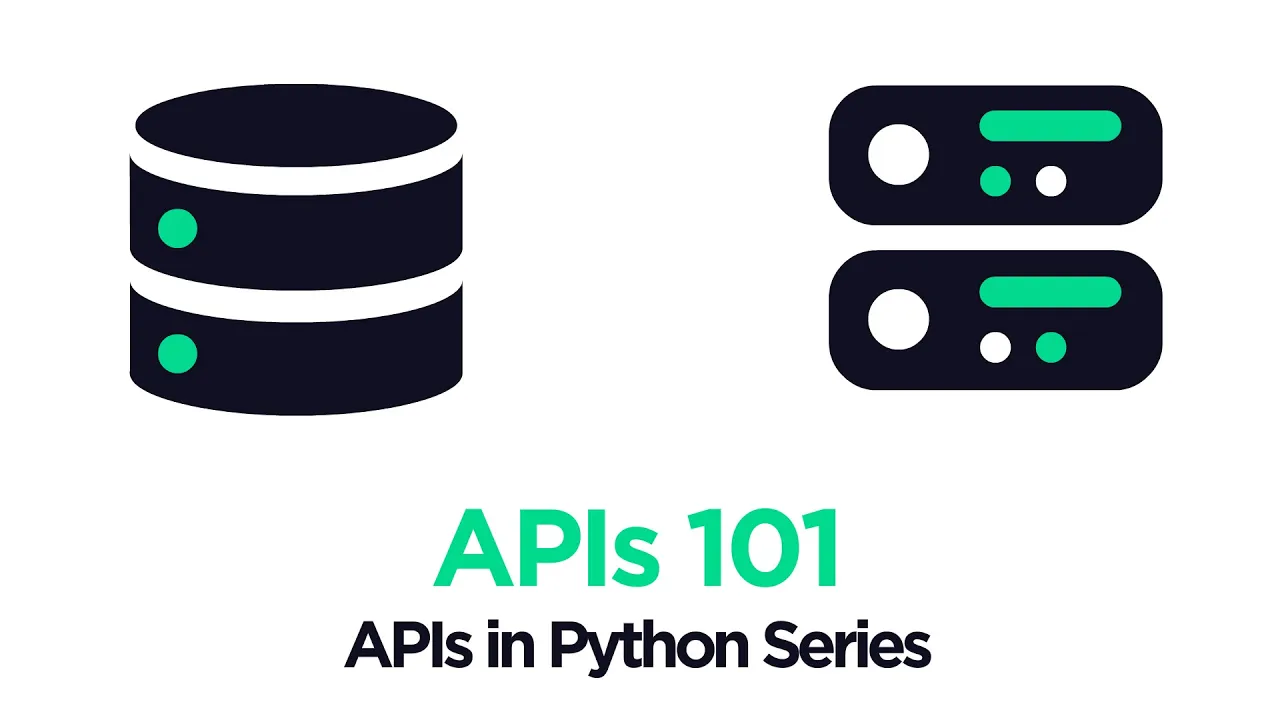
Chapters
0:0 Intro
0:20 What is an API?
1:47 RESTful APIs
5:26 API Methods
7:20 HTTP Codes (200s)
8:14 HTTP Codes (400s)
10:0 JSON Format
11:21 Talking to APIs in Python
14:30 Google Geocoding API
22:8 GitHub API
27:48 Outro
00:00:00.000 |
Hi, welcome to the video. We're doing something slightly different this time 00:00:03.920 |
We are going to put together a series of videos just covering 00:00:09.840 |
What apis are how we interact with them how we build them? 00:00:13.440 |
And we'll have a look at a few different python frameworks that we can use for putting them together 00:00:29.920 |
To start with stands for application program interface 00:00:37.920 |
So this thing in the middle here is our api now inside this magical black box 00:00:48.400 |
And all we really know is that it sends data back to us 00:00:57.280 |
This api here is a script on a server somewhere 00:01:03.680 |
What it is doing is acting as a middleman or a gatekeeper between us 00:01:09.040 |
And the server that it represents now through this api we can typically download or get information 00:01:17.380 |
We can request to change some information over on the server or we can request to delete information on server 00:01:26.160 |
And most requests we can kind of fit into one of those three things 00:01:30.320 |
We're either getting information updating or putting information or deleting information now 00:01:42.080 |
specific way of working so they generally all follow the same structure and the most common 00:02:00.700 |
Representational state transfer and that sounds really complicated in reality. It is very straightforward. We don't really need to 00:02:15.600 |
So we need to follow these six rules in order to make sure our api is RESTful or a REST api 00:02:26.480 |
Outward facing interface. So there's a single entry point 00:02:40.400 |
Like we saw before over here on the left. That is the internet and that is where we are coming from 00:02:51.920 |
And what this api does is acts as a middleman between both of those and it allows those two to 00:03:05.840 |
Connection between the client and server. We just all we need to do is communicate with the api 00:03:10.720 |
So our communications to the api should not change 00:03:14.000 |
Now if the server is updated and things change on the server the api 00:03:19.760 |
Should remain the same and therefore when we try to communicate with it. Nothing will change 00:03:28.000 |
What this means is say you send a api request 00:03:33.120 |
And then we send other api requests immediately after the results of that second api request 00:03:39.600 |
Should not rely or should not be dependent or change based on the results of the first request 00:03:47.440 |
unless of course we're changing information changing or deleting information and 00:03:52.400 |
Requesting all that information back again, of course in that in that case 00:03:57.200 |
It will change but the actual state of the api will not change 00:04:00.480 |
All that's changing there is the underlying data that is hosted on the server 00:04:05.520 |
Is changing but the state of the api does not change. It's a blank slate 00:04:16.160 |
For caching so down here all we're saying here is that the 00:04:23.120 |
It needs to inform us whether its responses are allowed to be cached by the user the 00:04:33.680 |
Api should follow a layered system structure. So there should be a modular structure to this api 00:04:41.440 |
So one layer can be changed and it should not affect the other layers 00:04:51.440 |
Should be able to provide code executable code on requests now, not all apis 00:04:58.160 |
Are relevant for this so we're not going to see this everywhere and as far as I know, it's reasonably rare as well 00:05:11.200 |
general users just means there'll be a single interface for us to interact with the api through 00:05:17.360 |
There are a specific set of behaviors that we would expect so we wouldn't expect this api to deviate too much 00:05:27.600 |
Now there are a set of different methods that we can interact with an api with 00:05:33.600 |
So we have a get request which is where we retrieve 00:05:38.220 |
Information a post request for creating a resource or creating basically creating a new record 00:05:45.340 |
On the other side of the api we have a put request for updating a resource or record 00:05:57.260 |
Now the most commonly used of these is the get request we use up 00:06:03.900 |
Quite a lot to be honest whenever you want information from an api you use a get request and then if you are 00:06:09.820 |
Interacting with the api in some way. There's a database that you're modifying behind it 00:06:13.980 |
You're going to use post put and delete quite a lot as well 00:06:20.700 |
gps coordinates from the google maps api we would use the get request so we'd be requesting 00:06:33.660 |
Alternatively if we were going to use the github api and we wanted to 00:06:39.500 |
Create a repository we wanted to update a repository or wanted to delete a repository 00:06:50.700 |
Now there is also another well, there are quite a few methods, but there is another 00:07:01.100 |
Um, so I mean the patch request is for partial updates 00:07:05.740 |
So it's like a put request but for partial updates now i've never actually seen this used so i'd 00:07:13.500 |
And if you use apis a lot, then maybe you'll use it 00:07:16.140 |
But I think for most users, you're probably not going to come across it 00:07:19.980 |
Okay. Now we've covered how we interact or what? 00:07:26.780 |
Requests we send to an api now. What about what it returns to us now? 00:07:31.340 |
An api will return different codes depending on the output 00:07:40.860 |
Result of whatever it is. We we asked it to do so 00:07:45.100 |
The first set of codes here are the 200 success codes. So anything the two 00:07:53.420 |
Anything within the 200 range is usually a successor. It's a good thing 00:07:59.660 |
We have the most common one, which is 200. Okay, which just means success in general 00:08:08.700 |
There's a success but the api didn't return anything. It's not necessarily a problem 00:08:17.180 |
The 400 codes now. These are client-side errors. So errors on our side 00:08:24.220 |
Actually to be honest. All these are pretty common 00:08:29.500 |
Means that you're probably doing something wrong is 400 is your bad request. That means we're entering the request wrong. So that might be 00:08:35.820 |
A the syntax is wrong the form the json format is wrong 00:08:45.180 |
Wrong fields in our request and then we have also unauthorized typically it's because we're not authorized ourselves with a authorization key 00:08:53.180 |
Forbidden so you're trying to access something that you're not allowed to access again 00:08:57.820 |
That might be because you're unauthorized and you just need to enter your auth key and then you'll be allowed there 00:09:04.060 |
And then also 404 which is not found that means there's nothing there 00:09:08.620 |
Although some websites like github will use this 00:09:13.900 |
When there is actually for example a repo there, but it's a secret and you're not allowed to see it 00:09:18.860 |
They'll give you a 404 because otherwise they're telling you. Hey, look, this is actually exist 00:09:23.180 |
So they they also give you that sometimes instead of forbidden or unauthorized 00:09:28.480 |
And then there's also these ones. So these are probably the two most important 00:09:33.920 |
Client error codes. So there's a 418 which is i'm a teapot 00:09:39.260 |
And and that's when you know, sometimes it happens where you ask a teapot to brew coffee 00:09:44.860 |
Um, so in that case just stop doing it and there's also 420 enhance your calm. So that's specific to twitter 00:09:51.820 |
And that's just saying you really need to chill and because you're sending far too many requests to twitter. Okay, and that's it for 00:10:02.220 |
The json object. So this is the format we use 00:10:06.780 |
For interacting with our api. It stands for json 00:10:14.140 |
And it I mean you can probably tell from this if you use python. It looks like a dictionary and it 00:10:19.020 |
Pretty much is a dictionary that they use the exact same structure 00:10:23.100 |
Although they're not technically the same but dictionary is a json like object 00:10:28.140 |
Now it's just a hierarchical format. It allows us to use all these different fields. We can put lists with strings 00:10:35.340 |
Put more dictionaries inside it. Uh, so it's it's pretty useful and this is the standard format. It's used by everyone 00:10:44.860 |
Now I can show you an example of this. So I just come over here. I have this little link here. So this is 00:10:50.700 |
Actually, we're going to go to an api in the browser and make a request from the browser 00:10:56.220 |
So we we can do that. It's not there's nothing weird about doing that and 00:11:04.380 |
To this it's called the pokey api. So it's actually an api for pokemon 00:11:10.220 |
Um, and it just returns you all this information. You can see that. I mean, it looks pretty messy 00:11:15.340 |
It's not on a clean format, but this is basically just a dictionary 00:11:21.660 |
Now, let's go back to our code and we're going to start putting something together. So we're going to start making some requests 00:11:29.900 |
So we first want to import requests, this is just a standard library for making api requests in python 00:11:37.260 |
So we just import requests and to make a get request. All we need to do is write request dot get 00:11:42.860 |
And let's use the the pokemon api that we saw before so i'm going to copy this 00:11:48.700 |
In fact, I already copied it. No, I didn't and so i'm going to copy this 00:11:56.940 |
And just bring it down here so enter is a string 00:12:03.500 |
I'm going to store this in the response variable there 00:12:07.100 |
And let's see what we get so we get a 200 response. So remember before 00:12:17.340 |
200 okay response so it's good. It means it went. Okay. It went well 00:12:22.460 |
Uh, but we that's all we see. So how do we actually access the the json response underneath that? 00:12:33.500 |
We use the json method and that's that's all there is to it 00:12:36.540 |
So now we see something very similar to what we've got in the browser before so we have this 00:12:41.020 |
Dictionary now, uh, we have these abilities. I'm not sure exactly what this is returning. I think it's just returning 00:12:49.340 |
Why is it returning? Oh, so we're searching for charizard 00:12:56.300 |
Has several abilities blaze solar power solar power 00:13:09.500 |
So if we want to access we can access abilities there 00:13:17.580 |
So now we need to access the first end level of that list and we have this other uh, this one entry here 00:13:29.100 |
Sort of a tree structure. We just keep going deeper and deeper in there 00:13:39.340 |
Just out of curiosity. I really want to see what what is there? 00:13:46.940 |
So it's just another is it is the api we can see that because it says up here i'll zoom in 00:14:07.980 |
Um, yeah, so we have that so maybe that's useful if you're really super into pokemon 00:14:16.000 |
But I don't know maybe like it for a game or something could be useful 00:14:19.360 |
I'm, not sure. I think to be honest. I think this is more for 00:14:26.720 |
Uh, it's pretty I mean it's pretty useful for this example. It's great 00:14:31.040 |
Now I want to show you something that's maybe more relevant 00:14:36.000 |
So i'm coming over to google and we have the google maps api 00:14:41.600 |
So documentation here just describes how you access it 00:14:45.200 |
You can you can follow it along if you want, but i'm going to very quickly just go through it 00:14:48.960 |
So we come down to create api keys go to credentials page. You'll probably need to create a project. So we click that 00:14:55.840 |
Uh doesn't matter what we call the project. So i'm just going to leave it with the default name we 00:15:03.680 |
Now you have to wait a minute for that to load 00:15:05.840 |
Okay, and then this page will load over here. So 00:15:10.320 |
What we want to do is we'll scroll down and we want to use the geocoding api. So we click there 00:15:20.960 |
Once that is loaded we want to head over here go to credentials 00:15:30.160 |
Okay, so i'm now just going to write that in here so we've got api key 00:15:41.760 |
Okay, I just need to make sure that is a code cell, okay 00:15:52.160 |
And then we also need a few other things so the api url so that is right out here 00:16:06.400 |
and then what we're going to do is we're going to enter an address and 00:16:12.960 |
Latitude and longitude from google maps. So what we need to do is write requests 00:16:21.120 |
Don't get because we're getting that information 00:16:34.400 |
And then we want to include the address that we want to search for so we go address 00:16:39.600 |
Equals and then in here we we write that so i'm going to go with the address for the coliseum in rome 00:16:58.960 |
Plus marks rather than a space if you you can't use spaces in 00:17:03.920 |
In http requests, so and you can also use this 00:17:17.120 |
And then the last thing in fact, actually, let me show you what happens if I try and run it like this. So let's 00:17:32.320 |
Okay, so we get 200 requests which is strange I would have expected 00:17:43.380 |
I would have expected a like a not unauthorized 00:17:54.000 |
So it's just saying so we return this error message saying you must use an api key, right? 00:17:59.360 |
Because we we have defined our api key up here, but we haven't included it in our request. So google doesn't know we actually have one 00:18:07.520 |
Uh, we also can include that so we come over here 00:18:25.360 |
Now let's have a look what we get so we will check we should still get a 200 response. It's good 00:18:31.920 |
And we also need to make sure that we write https there 00:18:44.960 |
all of the address information for the coliseum in room 00:18:49.040 |
and if we want so we said we wanted the longitude and latitude, so how do we get that we 00:18:58.960 |
We can see everything in there. So we have results or status 00:19:06.160 |
So, let me copy this come down so we have results and the results covers everything from here 00:19:26.560 |
So our results is is where we want to go for the longitude latitude because we have the latitude and longitude here 00:19:46.240 |
Components here and we want the first entry in that list 00:19:53.680 |
Okay, so it was just a it was a single entry in in the list 00:19:57.120 |
I assume they do that if you are requesting multiple addresses 00:20:03.360 |
And then we want to go into address components 00:20:05.380 |
Okay, and then we're in another list again here so we have this one 00:20:30.400 |
It's dictionary number five, I think. Yeah. Oh, no, no. No, um 00:20:34.640 |
Oh, we don't have we don't have the coordinates. So let me remove that. It's not address 00:20:58.400 |
Uh, I mean we can just actually no we can go for location. It's probably 00:21:04.640 |
Center I mean, I mean these are all obviously going to be very close. I don't think that 00:21:17.040 |
Okay, and then here we have our coordinates so we can we just save those to 00:21:38.960 |
Like so and there we are. We have our coordinates 00:21:48.240 |
I like to use that example because the the first sort of coding 00:21:52.160 |
Job, I ever had was using this weirdly enough 00:21:56.720 |
although the code back then was absolutely horrific when I 00:22:00.000 |
I I don't think I have it anymore. I'll have to have a look 00:22:03.600 |
Uh, but yeah, it was not very not very clean code. It's it's pretty horrific 00:22:09.360 |
And the next example, so we just that was an example of using the get request 00:22:14.960 |
but I want to show you also how we can use a put post or post put and delete as well, so 00:22:23.600 |
I think the easiest or most popular one that is just github. So 00:22:30.960 |
Come down here. So this is the documentation for getting access to it again link in description 00:22:40.480 |
We come to create a token. So i'm assuming you have a github account 00:22:46.320 |
So you just need to make sure everything is verified and it says you need to go into github 00:22:52.160 |
And click profile photo click settings. So we'll do that 00:22:56.720 |
Okay, so I have it open here come over here go to 00:23:05.760 |
And then over here on the left we want to go down until we see developer settings over here 00:23:10.960 |
And we want to go to this personal access token. So click on there 00:23:15.680 |
Uh, and then we just need to click on this generating token 00:23:19.840 |
Okay, and we just want to click on repo here. So it's all we need. Um, if you also want to delete i'm not going to 00:23:27.600 |
It's I think just a bad idea you can you can click delete repo here as well 00:23:35.760 |
And then I have it down here. So i'm going to copy that over into my notebook 00:23:40.980 |
Okay, so i've put that in a variable called github key 00:23:49.280 |
Create a a new repository. So we're going to send a post request 00:23:55.740 |
Initialize a new repository. Um to do that. We we also need this other 00:24:00.000 |
Library, so import json. I'll show you why in a moment 00:24:09.920 |
We'll see in a moment, but i'm going to create this dictionary which is going to contain information for my api requests 00:24:17.120 |
So typically you you would you use this when you're using? 00:24:25.600 |
Think as well. So you generally you are going to use this but not so much for get requests 00:24:31.920 |
So you have the name of the repository which is going to be api test 00:24:36.720 |
And i'm also going to set it to be public so we can 00:25:01.840 |
And then in the headers so we have this other 00:25:08.960 |
sort of your authorization typically and then any other 00:25:16.160 |
Which is what we're going to use it for so we're going to use it to include our authorization code 00:25:20.800 |
and you'll typically find that that is formatted in in 00:25:25.760 |
Slightly different ways. It's not just a string. You also need to include something else 00:25:41.040 |
I need to put token space and then in here I have my my actual token. So github 00:25:52.960 |
And then this is where we include our payload and why we're using the json 00:26:05.840 |
convert our dictionary here into a json formatted string 00:26:10.720 |
Okay, because we can't just include a dictionary in a json request. You you will get you'll get an error 00:26:17.680 |
So you do need to convert that into a json json string 00:26:26.800 |
That looks pretty good. So let's let's try it 00:26:28.880 |
Let's see we got we got a 422 response, so let's see why 00:26:40.300 |
Creation failed because I already have it. I've already tested it before apparently so 00:26:44.560 |
I'm, not very creative with my names. So we're going to call it api test 2 00:26:54.560 |
Uh, but notice with with that, uh response that we although we haven't covered 422 00:26:59.860 |
Uh, we could see that was a 400 code. So we knew that something had gone wrong on our side 00:27:18.880 |
New repository. So if I go to my github and have a look I should see that 00:27:28.000 |
And right at the top there. We see this api test 2 so we did create it 00:27:34.160 |
And from now we can do other things you can you can post things to your repos. You can delete them 00:27:42.080 |
You just need to modify which permissions you've set when we build up when we create that personal access token 00:27:53.680 |
I think we've covered quite a lot. We so we've covered the essentials of apis had a quick look at the pokemon api 00:28:03.840 |
Google maps geocoding api and now the github api so 00:28:10.960 |
Video what I want to do is have a look at how we can build an api 00:28:19.440 |
So, thank you very much for watching and I'll see you in the next one. Bye!