back to indexSequential Model - TensorFlow Essentials #1
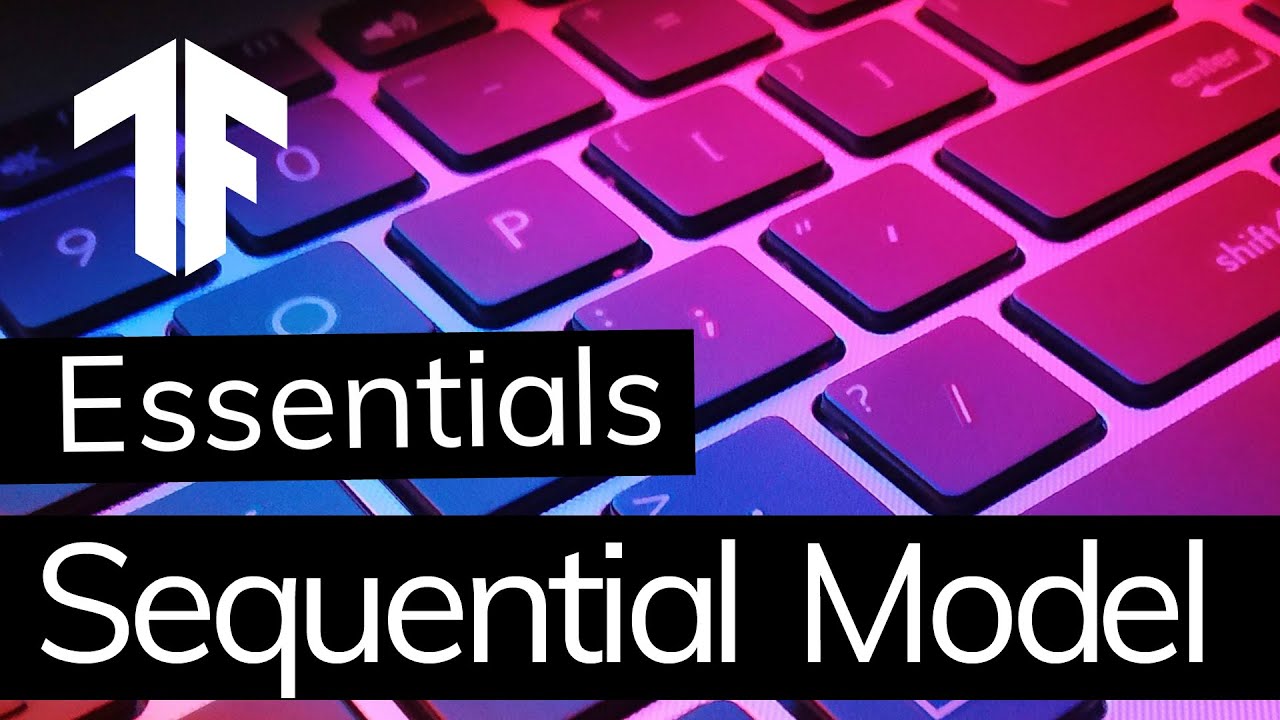
Chapters
0:0
0:7 start a new notebook
2:54 add another layer
3:44 using the softmax activation
4:27 use the softmax activation function
4:36 print out our model with the model summary
5:23 building a sequential model
00:00:00.000 |
Hi, welcome to this introduction to the sequential model in TensorFlow. 00:00:04.800 |
So we're just going to go ahead and start a new notebook here. 00:00:12.800 |
Now, the sequential model is one of two different approaches 00:00:21.600 |
that we can use for building our models in TensorFlow. 00:00:27.400 |
and then the sequential model that we're going to go through here. 00:00:30.600 |
So the sequential model is better for simple sequential stacks of layers 00:00:35.600 |
where each layer has just one input and just one output. 00:00:43.400 |
then the sequential approach is probably better. 00:00:49.500 |
So we access the sequential model using tf.keras.sequential 00:00:58.600 |
And then that initializes the sequential model. 00:01:07.700 |
And now there are two methods for adding layers with this model. 00:01:12.000 |
The first of those which I'm going to show you is using the add method. 00:01:16.800 |
So all we do is take model, add, and then we add our layer here. 00:01:24.100 |
So one of the layers that we'll be using quite a lot 00:01:37.000 |
And then we're going to add in 32 units here. 00:01:50.300 |
and they are each connected to 32 units within our dense layer here. 00:02:04.200 |
Now you don't need to know all of this right now 00:02:08.100 |
but what I do want you to focus on is the shape that we are producing. 00:02:16.700 |
this is the shape of the network that we're building. 00:02:27.000 |
And then we have 32 neurons in the middle, so 32 units. 00:02:31.900 |
If you look, because it's a densely connected neural network, 00:02:35.100 |
every single one of these is connected to every single one. 00:02:38.500 |
So all of our 10 inputs are connected to this unit here, 00:02:46.300 |
And then in our output, I want to put 2 units. 00:02:52.500 |
So if we want to add another layer, we just call add again. 00:03:02.800 |
And if we want to add another layer, all we do is model add again. 00:03:14.800 |
in our first layer with the sequential model. 00:03:17.800 |
Otherwise, we don't need to define it because it will assume 00:03:21.300 |
that our input shape matches the shape of the previous layer, 00:03:28.200 |
So we just put 2, and then we add in our activation. 00:03:48.700 |
So in the output layer, you will usually have either sigmoid or softmax. 00:03:58.100 |
so if your output is just one value between 0 and 1, 00:04:06.700 |
If you have multiple outputs, so we have two units here, 00:04:10.000 |
that means our output can look something like this. 00:04:12.900 |
And what we will want to do is take the maximum value 00:04:28.500 |
we want to use the softmax activation function. 00:04:53.800 |
And then it goes into the actual model, which is 32 units. 00:05:01.300 |
And then our output layer is two units at the end there. 00:05:05.600 |
And we see that there are a total of 418 parameters 00:05:15.700 |
in order to learn patterns within the training data. 00:05:34.600 |
all within the single initialization function 00:06:14.900 |
So that is everything on the sequential model. 00:06:22.000 |
I hope you've enjoyed and I hope it's been useful. 00:06:24.900 |
So thank you for watching and I will see you again soon.